C# generation commands
Modelio C# Designer generation provides several features related to generation.
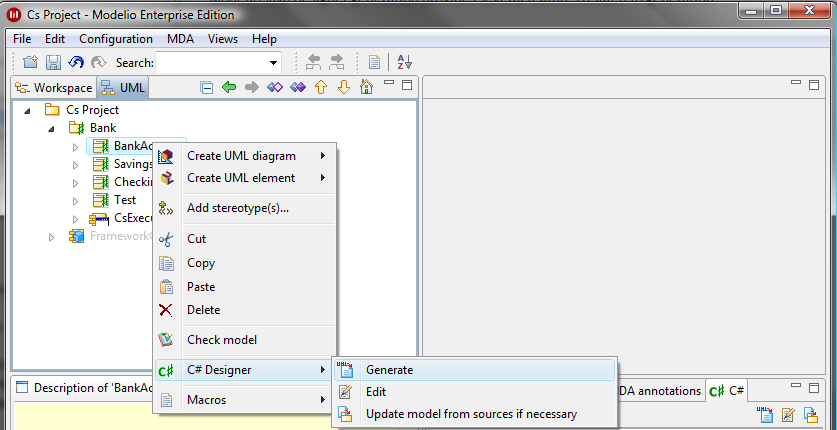
List of commands:
-
Generate: Generate C# code for the class.
-
Edit: Open an editor to modify the C# file content. When the editor is closed, the content of the file is automatically reversed.
-
Update model frome sources if necessary: Reverse the C# code for the class.
Defining C# attributes
C# attributes are defined through the C# property view of a Class, by choosing ‘C# Attribute’ value in the C# element field.
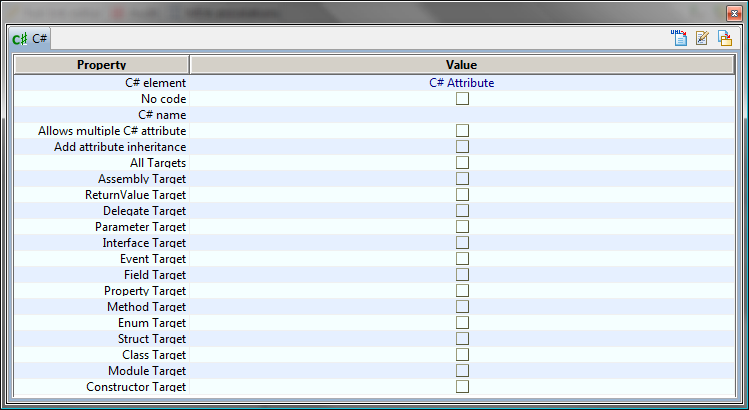
This view defines all options available on the C# Attribute definition.
-
Allows multiple C# attribute: Specifies whether or not the multiplicity of the C# attribute is allowed.
-
Add attribute inheritance: Specifies whether or not the C# attribute is inherited.
-
Attribute targets: Specifies the types of elements where the Attribute is applicable.
Defining C# indexers
Indexers are modeled through a UML class with two operations, "get" and "set", to which the «CsIndexer» stereotype is added. A class stereotyped «CsIndexer» will not be generated. It will simply be used to generate C# indexer get and set signatures and bodies.
To create an indexer, simply use the ‘Create C# Designer element’ contextual menu on a class:
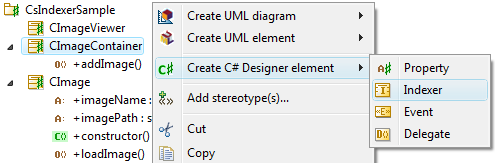
The following is a description of indexer accessors:
-
"get": The "get" operation has parameter(s). This (these) parameter(s) allow the indexed research in your array.
-
"set": The "set" operation has at least two parameters, and must have the value to set for the right-hand parameter.
These functions help to resolve the indexer signature. Indexer signatures are as follows:
Indexer-visibilityindexer-returnType this[indexer-parameters] { ... }
-
indexer-visibility is defined by the class visibility.
-
indexer-returnType is defined by the "get" operation return parameter or by the "set" operation right-hand parameter.
-
indexer-Parameters are defined by the "get" operation parameter(s) or by the "set" operation parameters except the right-hand one, corresponding to the value to be defined.
Defining C# events and delegates
C# events are modeled throught a UML signal to which the «CsEvent» stereotype is added. This signal must be a part of a class. Once a signal is stereotyped as an event, you can manage new values such as visibility (using the C# property view) and delegate association (using Signal UML properties tab’s Base field). An event must refer to a model’s delegate.
To create a C# event, simply use the ‘Create C# Designer element’ contextual menu on a class:
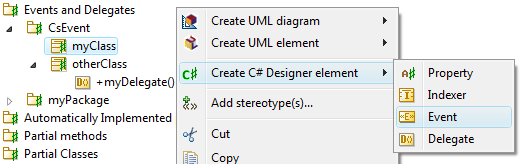
Delegates can be operations issued from C# delegate containers (classes stereotyped «CsDelegateContainer») or C# delegates (operations stereotyped «CsDelegate»). The related reference can be created through a drag and drop operation.
C# delegate containers (classes stereotyped «CsDelegateContainer») are used as containers of C# delegate types (operations stereotyped «CsDelegate»). The generated delegates belong to the current parent element.
Stereotyping an operation «CsDelegate» will enable delegate signature and skip generation of all code notes attached to the operation.
Defining C# automatically implemented properties
To obtain the property declaration with simplified syntax, you must not define any associated code notes (CsGetCode/CsSetCode), as if you do, a normal property will be generated.
The generator will automatically apply the "private" modifier (by default) to the get or set accessor according to which access mode has been selected, as shown in the table below.
Access mode / Modifiers | None | Read | Write | ReadWrite |
---|---|---|---|---|
get |
Error |
Warning |
CsPropertyVisibilityGet or private |
Warning or Error |
set |
Error |
CsPropertyVisibilitySet or private |
Warning |
Warning or Error |
Example of automatically implemented properties
-
UML Model
-
C# Generated
using System; using System.Diagnostics; namespace ExtensionMethods { public class LightweightCustomer { #region TotalPurchases public int TotalPurchases { get; set; } #endregion #region Name public string Name { get; private set; } // read-only #endregion #region CustomerID public int CustomerID { private get; set; } // write-only #endregion } }
Defining C# partial methods
To define a method as being a partial method, simply check the "Partial" tick box in the C# property view. Modelio C# Designer will then position the new "partial" modifier just before the void keyword during code generation.
The generator will ignore the {CsPartial} tagged value if the method has a return parameter, one or more "out" parameters, or a virtual, abstract, override, new or sealed modifier. Furthermore, the visibility of the method must be private or default (implicitly private).
Example of using partial types and methods
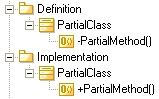
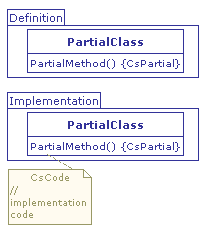
// Definition in file1.cs using System; using System.Diagnostics; namespace Project.Definition { public partial class PartialClass { partial void PartialMethod(); } } // Implementation in file2.cs using System; using System.Diagnostics; namespace Project.Implementation { public partial class PartialClass { public void PartialMethod() { // implementation code } } }
Note 1: A partial method containing a "CsCode" or "CsReturned" note will generate the implementation part. The definition part will be produced if the partial method does not have these notes.
Note 2: A partial method declaration is made up of two parts: the definition and the implementation. It is up to the user to only create a single definition/implementation couple.
Be careful, Modelio C# Designer runs no tests on this.