Introduction
Generics are a new functionality provided by version 2.0 of the C# language and the common language runtime (CLR). Generics introduce the concept of type parameters into the .NET Framework, making it possible to receive classes and methods whose specification of one or several types differs until the class or method is declared and instanciated by client code.
The "SDK Version" tickbox (contained in the "General" parameter set, as shown in the screenshot below) tells Modelio C# Designer whether or not this new functionality (and the other .NET Framework evolutions) is available during code generation.
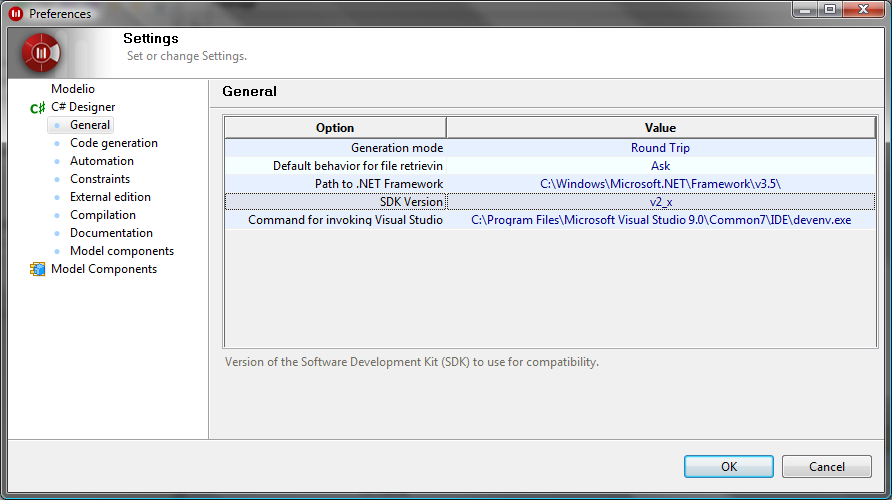
Note: Do not modify the .NET Framework directory containing the version number during .NET installation.
Generic collection classes
An additional namespace, "System.Collections.Generic", has been added to the Framework 2.0. This namespace contains the interfaces and classes that define the generic collections used to create strongly typed collections.
-
Dictionary: Represents a collection of keys and values.
-
LinkedList: Represents a double-link list.
-
List: Represents a list strongly typed by objects accessible through the index. Provides search, sort and handling methods for lists.
-
Queue: Represents a collection of objects, first in, first out.
-
SortedDictionary: Represents a collection of key/value pairs sorted by key.
-
SortedList: Represents a collection of key/value pairs softed by key according to the associated Icomparer implementation.
-
Stack: Represents a first in first out-type collection of variable size of instances of the same arbitrary type.
Modelio C# Designer provides and uses these new collections instead of the earlier ones, as they provide better security and performance than non-generic strongly typed collections (see below).
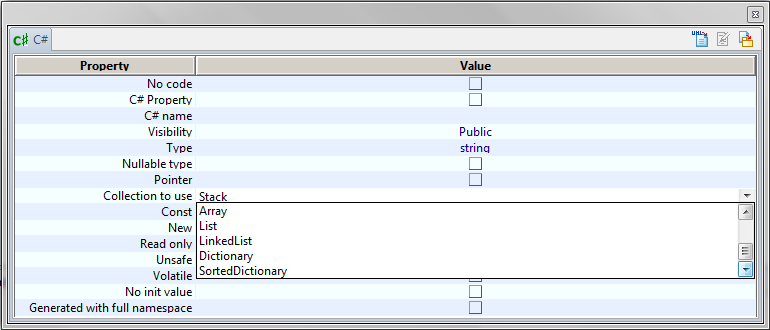
Note: The "List" generic collection is used by default.
UML model | Generated C# |
---|---|
If Modelio C# Designer uses the .NET Framework 2.0, then associations and attributes whose multiplicity is greater than one will be generated using generic collections, as far as possible (see the table above).
Generic classes, methods and delegates
To make your class, method or delegate generic, add a template parameter in the explorer.
The following table shows an example of a generic class and the generated C# code.
UML model | Generated C# |
---|---|
The following table shows an example of a generic method and the generated C# code.
UML model | Generated C# |
---|---|
The following table shows an example of a generic delegate and the generated C# code.
UML model | Generated C# |
---|---|
Template parameters and constraints
When you are defining a generic class, you can apply certain restrictions to the kinds of types that the client code can use as type arguments when it instantiates your class. These restrictions are called constraints. The following table presents the six types of constraint that exist:
List of constraint:
-
where T : struct: The type argument must be a value type. Any value type, except Nullable, can be specified.
-
where T : classe: The type argument must be a reference type, including any class, interface, delegate or table type.
-
where T : new(): The type argument must have a constructor without public parameters.
-
where T : <base class name>: The type argument must be or derive from the specified base class.
-
where T : <interface name>: The type argument must be or implement the specified interface. Several interface constraints can be specified. The interface that imposes the constraints can also be generic.
-
where T : U: The type argument provided for T must be the argument provided for U, or must derive from it. This is what is called a naked type constraint.
Note: Only the "BaseClass" constraint (base class name) must be indicated in the template parameter UML properties view (see the "Generic classes, methods and delegates" paragraph above). All the other are added using a tagged value (see "Tagged values types").
The table below shows the intercompatibility of constraints:
-
No [ 1 ]: The constraint can only be present once
-
No [ 2 ]: The type argument cannot simultaneously be a valued type and a reference.
-
No [ 3 ]: The reference cannot be specialized (BaseClass) and general (class).
struct | class | new() | <baseclass> | <interface> | U | |
---|---|---|---|---|---|---|
struct |
No[1] |
No[2] |
No[2] |
No[2] |
Yes |
Yes |
class |
No[1] |
Yes |
No[3] |
Yes |
Yes |
|
new() |
No[1] |
Yes |
Yes |
Yes |
||
<baseclass> |
No[1] |
Yes |
Yes |
|||
<interface> |
Yes |
Yes |
||||
U |
Yes |
The following table shows an example of a generic class with a "BaseClass" type constraint.
UML model | Generated C# |
---|---|
The following table shows an example of a generic method with an "Interface" type constraint.
UML model | Generated C# |
---|---|
Specifying type arguments
To specify a type argument, a concrete (or generic) type must be associated to: the attribute, parameter, role or inheritance link. In the explorer, select the element in question and then in the MDA annotations view, associate the {Bind} tagged value, with a types list as parameter (see the screenshot below).
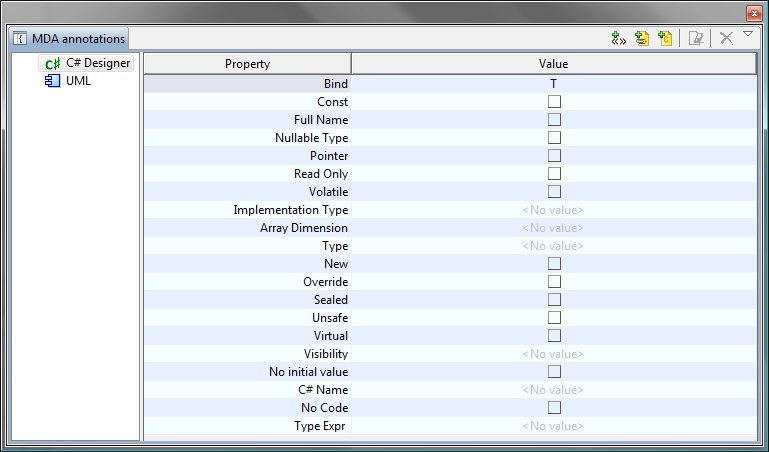
The following table shows an example of the association of a generic class with a parameter left unspecified ("T").
UML model | Generated C# |
---|---|
The following table shows an example of an inheritance link: closed constructed type.
UML model | Generated C# |
---|---|
The following table shows an example of an inheritance link: open constructed type.
UML model | Generated C# |
---|---|
The following table shows an example of a generic parameter of a method left unspecified ("T").
UML model | Generated C# |
---|---|