The automatic decoration flag
By default, Modelio Cxx Designer automatically deduces Cxx decorations for parameters, attributes and association ends. However, in certain cases, deduced decorations may not correspond to what you actually intended. For example, you may want to override an externally defined virtual function, whose prototype does not correspond to that deduced by Modelio Cxx Designer.
Modelio Cxx Designer lets you mark the model elements to be avoided by the automatic deduction procedure. For this, the "Cxx" property view contains the "Automatic Decoration" tickbox.
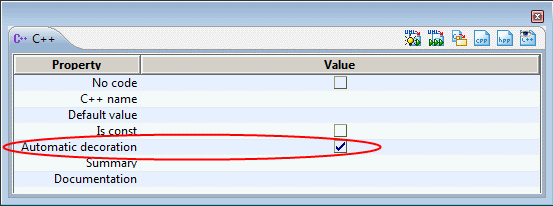
By default, the automatic decoration flag is always on.
By switching off the flag, you indicate that the selected element should not be taken into account in the automatic deduction process. In this case, Modelio Cxx Designer only takes in account your decorations, and does not extrapolate new ones. To help you, deduced decorations are saved into the model, so that you can use them as a basis.
By switching on the flag, you indicate that the selected element is to be taken into account during the automatic deduction process. In this case, any decorations you have previously created are removed, and Modelio Cxx Designer deduces new decorations at generation according to the rules defined.
Note: The automatic decoration strategy is also called automatic guessing.
See the corresponding section in the advanced part of this guide for more information.
Note 2: Editing Cxx properties in the corresponding edition box sets the automatic decoration to off.
Automatic decoration of structural features
Structural features are attributes and associations between UML classes. Many existing Cxx generators translate these as C pointers. However, C pointers are a poor choice to represent the semantics of relationships between model elements. Consequently, you have to implement this at code level and the model then diverges from code, or else you are distracted by low-level details, such as collection types for multiple attributes, storage by pointer or by value, and so on.
Modelio Cxx Designer lets you focus on the high-level properties of application entity relationships and attributes. It automatically deduces Cxx decorations, which specify collection and storage type, by analyzing the high- level properties of structural features (multiplicity, uniqueness, ordering, and qualifying attributes). These properties are often used to represent semantics on UML models.
We are now going to continue by creating a more detailed model of our
application prototype.
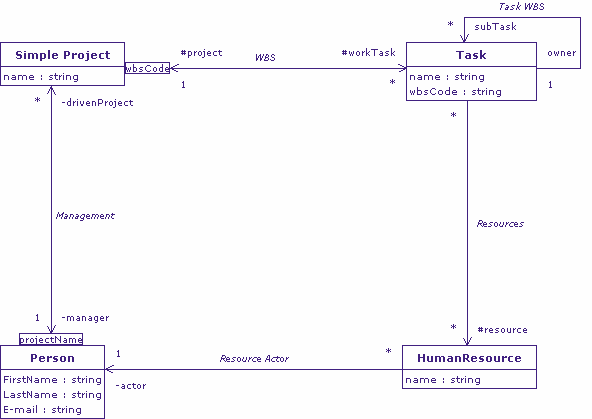
The "Management" association links a "Person" to a "SimpleProject". One manager can drive several projects, and there is only one manager for each project, so multiplicity is set to "1" and "*". A manager can drive a project only once, and THE order of projects is not important, and we therefore specify uniqueness and no ordering for the "drivenProject" association end. For rapid access to a driven project, we qualify projects by project names, which are used as project keys.
By analyzing these properties, Modelio Cxx Designer deduces that the "manager" association end is represented by simple pointer, and that the "drivenProject" association end is represented by "UnorderedMap". Unordered maps are naturally represented by hash map collections, so Modelio Cxx Designer automatically creates the respective declarations.
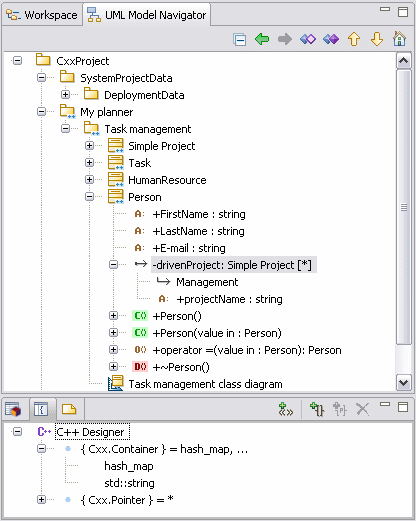
In our example, an STL-type library is chosen by default. Consequently, Modelio Cxx Designer produces the following definition of the "Person" class.
class Person { //... public: std::string FirstName; std::string LastName; std::string Email; //associations private: std::hash_map<std::string,SimpleProject> drivenProject; //operations public: Person(); Person(const Person& value); Person& operator =(const Person& value); ~Person(); //non-modeled members };
Automatic decoration of operation parameters
Since Cxx does not provide automatic memory management capabilities, you as a Cxx user have to pay particular attention to the method you use to pass operation parameters, in order to avoid producing inefficient or incorrect code. For example, if a class parameter is passed by value, a copy constructor will be called, and the operation code will modify the copy, not the object itself, and so on.
Modelio Cxx Designer lets you focus on high-level UML model properties without being distracted by low-level details, such as passing operation parameters by reference or by value or const qualifiers for "in" parameters.
Modelio Cxx Designer analyzes a parameter’s type and sets the "by reference" passing modes for class or datatype parameters, as well as for parameters of non-primitive types defined in type libraries. In Cxx, this mode is implemented by adding a reference specifier to the parameter type. Modelio Cxx Designer adds const specifiers to "in" parameters in order to explicitly express the fact that these parameters cannot be changed by the operation body, and const specifiers to "in" operations in order to explicitly express the fact that these operations do not change object state.
Consider our application prototype model. Continue by creating the "addSubTask" operation in the "Task" class operation, which takes a subtask to add and its number in the task work breakdown structure.
Before generation, Modelio Cxx Designer automatically creates Cxx decorations for its parameters. It adds a reference specifier to the type of the "SubTask" parameter, since this type is a class. It does not add a const specifier to this parameter, because it has "inout" semantics and can therefore be changed by the operation. It does not create a const specifier for the "wbsNum" parameter, because it is of primitive type. The integer type is translated to the Cxx int type, which is passed by value, so there is no point in qualifying the "wbsNum" parameter as a const.
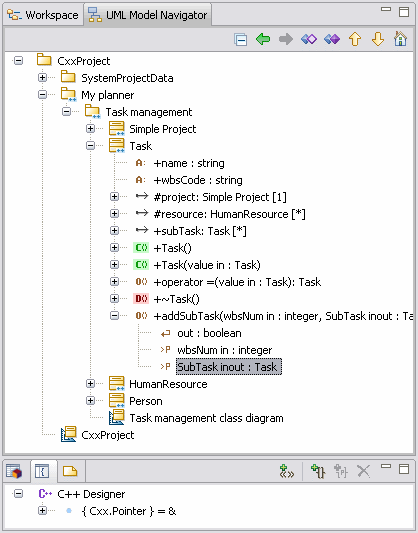
Modelio Cxx Designer produces the following declaration of the "addSubTask" operation.
class Task { //... public: std::string name; std::string wbsCode; //associations protected: SimpleProject* project; std::set<Task> subTask; Task* owner; std::list<HumanResource> resource; //operations public: Task(); Task(const Task& value); Task& operator =(const Task& value); ~Task(); bool addSubTask(int wbsNum, Task& SubTask); //non-modeled members };