The methods used to access the attributes of application classes constitute an important part of model semantics. However, these accessors are traditionally produced only at code level, with the result that the model differs from the code.
Modelio Cxx Designer is the first solution to support the automatic creation of model-level accessors for structural features.
To create accessor operations for an attribute or association end, simply select the element in question and change its access mode from the UML property or the Cxx Designer view.
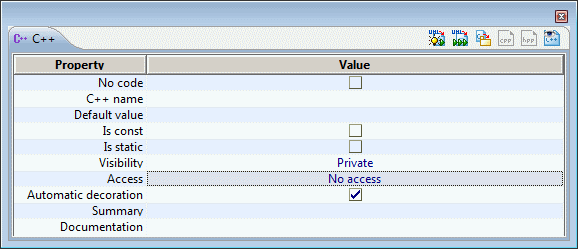
Modelio Cxx Designer implements accessors through design patterns. The current accessor pattern creates the "get" accessor operation if the feature has a "read" access flag (which corresponds to get access), and the "set" accessor operation if the feature has a "write" access flag (which corresponds to set access).
For a single cardinality primitive-type attribute, the get operation returns the attribute and the set operation sets its value. For an association end with single cardinality or a complex-type attribute, the get operation returns the reference to the target class and the set operation sets the feature value from a given const reference. For implemented collections with multiple cardinality features, get and set accessors are implemented by a single operation that returns the reference to the collection instance, which is a const if the "write" (set) access mode is not specified.
As an example, let’s run the "Create accessors" command on the "subtask" association end in the "Task" class, which has "*" multiplicity and read-only access semantics expressed by only the "read" (get) access mode. Modelio Cxx Designer will then create the UML operation "getSubTask" in the "Task" class.
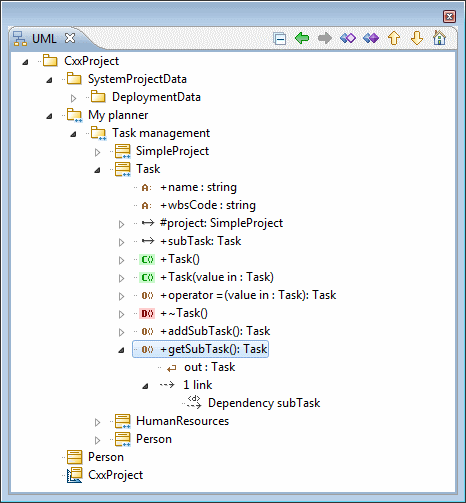
Modelio Cxx Designer copies the Cxx decorations (that were automatically deduced for the "subtask" association end from its uniqueness and ordering properties) to the return type of the created accessor, additionally decorating it with the const specifier to represent read-only semantics. Modelio Cxx Designer also creates the Cxx code note, which implements default accessor implementation.
Modelio Cxx Designer produces the following code for the "Task" class, which implements get access to the "subTask" member.
class Task { //... public: std::string name; std::string wbsCode; //associations protected: SimpleProject* project; std::set<Task> subTask; Task* owner; std::list<HumanResource> resource; //operations public: Task(); Task(const Task& value); Task& operator =(const Task& value); ~Task(); bool addSubTask(int wbsNum, Task& SubTask); const std::set<Task>& getSubTask() const; //non-modeled members }; const std::set<Task>& Task::getSubTask() const { //modifiable zone @12953@30671900:493@T return subTask; //modifiable zone @12953@30671900:493@E }
Model-level accessor management is dynamic, meaning that when an attribute is updated, its accessors are automatically updated too. If you rename an element, its accessors are automatically renamed. If you change an element’s access mode, the respective accessors are automatically created or deleted, and the const specifiers of collection accessors are automatically created or removed.
Generation of accessors directly at UML model level and their automatic synchronization with accessed elements is used to conveniently express access semantics at a high level, thereby creating a closer link between the application model and the code, starting from the prototyping stage.