Introduction
A type library groups together type definitions, which guide the translation of primitive types, data types and collection types used when modeling Cxx implementations.
Selecting a type library
A type library can be selected on two levels, a project build targets or individual structural features or parameters.
To set a type library for a chosen model element, you simply select it in the library selector in the element’s Cxx edition dialog box.
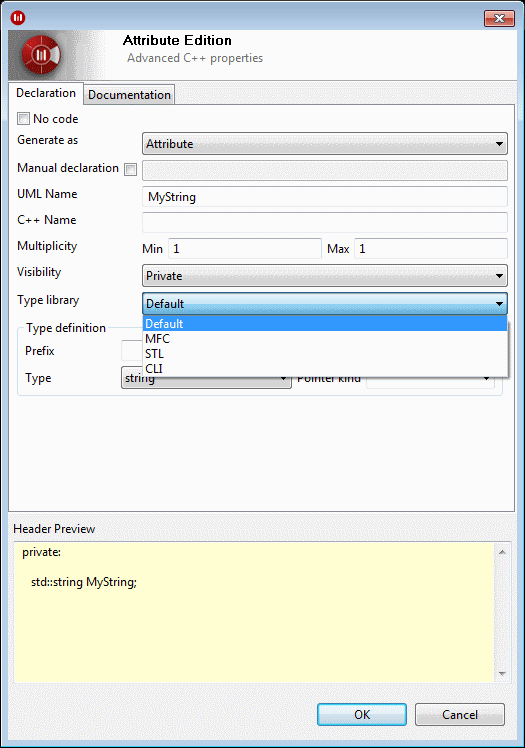
Modelio Cxx Designer automatically uploads new type libraries and visualizes them in the library selector. The "Default" choice uses the current project’s type library for generation.
Let’s consider a more detailed model of our task management application. First we model the application GUI for the Windows platform, which uses the MFC library to implement GUI functionalities. We then design the "TaskWindow" class, which represents the window used to graphically visualize of task information (owner, subtasks, resources, and so on).
Let’s say that this class has the "Brush Resources" association with the "CBrush" class, which represents the display (GDI) brushes used to render tasks of various different statuses. To rapidly access brush resources, brush instances are associated with brush names.
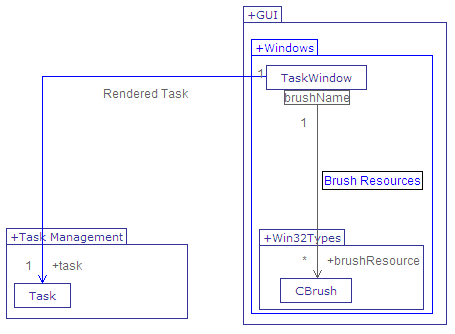
We will now continue by selecting the "MFC" type library for the current project.
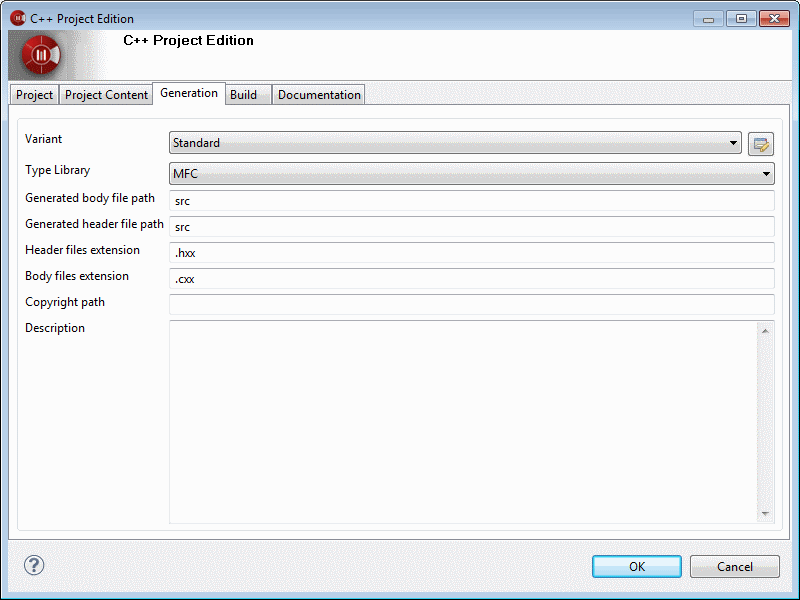
As a result, Modelio Cxx Designer produces the following code for the "TaskWindow" class. The "OrderedMap" semantics of the "browserResource" association end correspond to the "map" collection type provided by the MFC type library and are implemented by the "CMap" type. The include directives required to use this type are automatically generated.
//includes for used library types #include <afxcoll.h> //automatic includes (friends, associated classes, etc) #include "MyPlanner/TaskManagement/Task.h" //modifiable zone @13936@30671900:989@T #include "afxwin.h" //modifiable zone @13936@30671900:989@E namespace MyPlanner { namespace GUI { namespace Windows { class TaskWindow { //... public: TaskManagement::Task* task; CMap<CString,CString&,CBrush,CBrush&> brushResource; //operations public: TaskWindow(); TaskWindow(const TaskWindow& value); TaskWindow& operator =(const TaskWindow& value); ~TaskWindow(); //non-modeled members }; } } }
Multiple type libraries
It is possible to specify type libraries up to particular structural features and parameters, thereby making it possible to have multiple type libraries defined for members of a single class.
Let’s continue by modeling the fact that the "TaskWindow" class implements the "ITaskView" interface, designed to support cross-platform GUI. This interface uses the "STL" type library due to STL portability.
The "ITaskView" interface defines the "formatDisplayTitle" operation used to format the task display title according to a given formatting string.
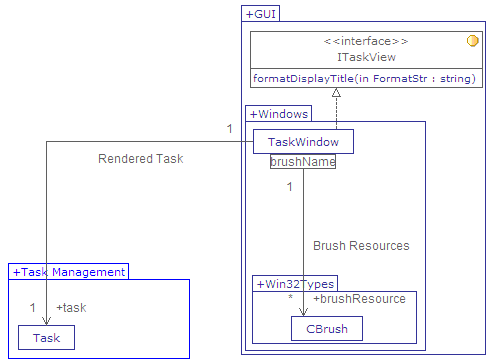
We are now going to create the string type "displayTitle" attribute in the "TaskWindow" class, which represents the task title rendered in the window. In this class, we will implement the "formatDisplayTitle" operation from the "ITaskView" interface.
If we simply generate the code, the "formatDisplayTitle" function defined in the "TaskWindow" class will not override the interface operation, because its string parameter type will be translated to the "CString" type, which implements it in the "MFC" type library.
To make the operation override the interface method, we will now specify the "STL" type library individually for its parameter.
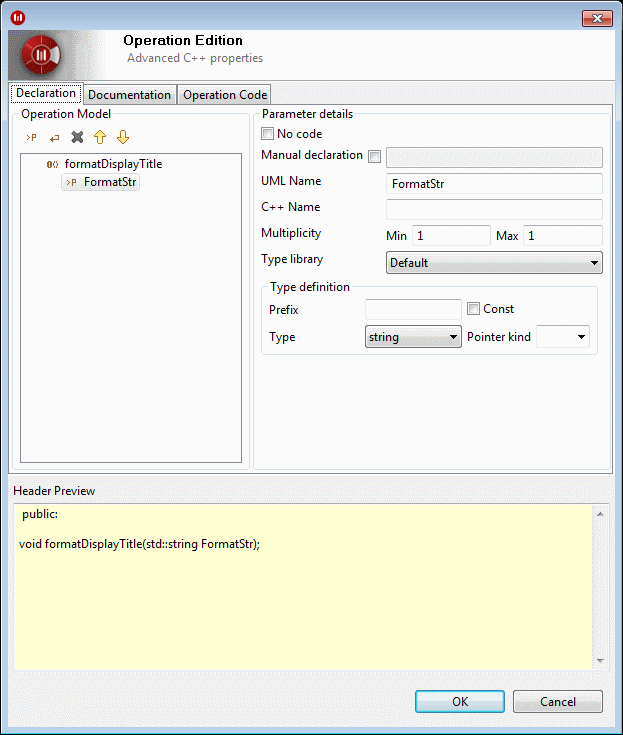
Modelio Cxx Designer now produces the following code for the "TaskWindow" class. Implementations of string types and include directives for their headers from both the "MFC" and "STL" type libraries are presented.
//includes for used library types #include <cstringt.h> #include <string> #include <afxcoll.h> //automatic includes (friends, associated classes, etc) #include "MyPlanner/GUI/ITaskView.h" #include "MyPlanner/TaskManagement/Task.h" //modifiable zone @13936@30671900:989@T #include "afxwin.h" //modifiable zone @13936@30671900:989@E namespace MyPlanner { namespace GUI { namespace Windows { class TaskWindow : public GUI::ITaskView { //... private: CString displayTitle; //associations public: TaskManagement::Task* task; CMap<CString,CString&,CBrush,CBrush&> brushResource; //operations public: TaskWindow(); TaskWindow(const TaskWindow& value); TaskWindow& operator =(const TaskWindow& value); ~TaskWindow(); void formatDisplayTitle(std::string FormatStr); //non-modeled members }; } } }
Note 1: You can also create your own type libraries using Modelio Cxx Designer platform development features, by editing open ACT type library definitions. Type library selectors are fed dynamically, so when a new type library is installed, it is immediately visible and available for selection.
Note 2: When a type library is selected for a particular model element, this only suggests that it be used for type translation, but does not force Modelio Cxx Designer to use only type implementations from this library. Therefore, when the type or collection type of a model element cannot be translated with a type library selected for it, the type library selected for its owner applies.
Using non-primitive types
A type library can define non-primitive types, which are not semantically compatible with basic UML types, such as string or integer. To use such a type, create an empty datatype with the same name in the model, and then assign this datatype to the attribute or parameter type in the usual way. Modelio Cxx Designer automatically substitutes it for the library definition, inducing include directives and applying ACTs specified there.
It can be useful to create these data types in a dedicated package specified not to produce the namespace and not to produce Cxx code.
For example, the "MFC" type library provides the "CDC" type expressing the respective "MFC" class, which represents the device drawing context.
Create the "dc" attribute used to store the device context to draw the task window in the "TaskWindow" class. This attribute should have the "CDC" type.
It would not be wise to model the "CDC" class from MFC, because it defines a huge number of member functions. In order to use the CDC type defined in the "MFC" library, create the "CDC" datatype in the dedicated "Win32Types" package, marked as no code and no namespace.
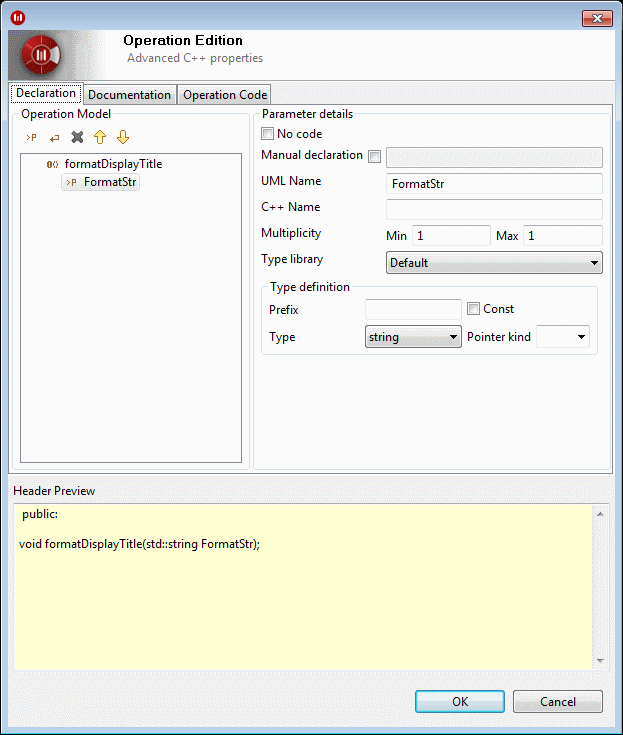
Please note that the data type has been automatically decorated with the «Cxx DataType» stereotype.
Continue by assigning the "CDC" datatype as being the type of the "dc" attribute in the "TaskWindow" class, by dragging it into the "Class" field in the standard attribute dialog box.
Modelio Cxx Designer produces the following code for the "TaskWindow" class. Translation of the datatype to the "CDC" class and include directives required to use this class are presented.
//includes for used library types #include <cstringt.h> #include <string> #include <afxwin.h> #include <afxcoll.h> //automatic includes (friends, associated classes, etc) #include "MyPlanner/GUI/ITaskView.h" #include "MyPlanner/TaskManagement/Task.h" namespace MyPlanner { namespace GUI { namespace Windows { class TaskWindow : public GUI::ITaskView { //... private: CString displayTitle; CDC dc; //associations public: TaskManagement::Task* task; CMap<CString,CString&,CBrush,CBrush&> brushResource; //operations public: TaskWindow(); TaskWindow(const TaskWindow& value); TaskWindow& operator =(const TaskWindow& value); ~TaskWindow(); void formatDisplayTitle(std::string FormatStr); //non-modeled members }; } } }